Help message
Lanat provides a way to automatically generate help messages for your commands. Specifying the descriptions in the command and its arguments is usually enough to generate a rich and helpful help message.
As an example, this is how a simple argument with a description can look like:
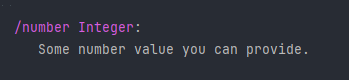
Here's how a command with more arguments with rich descriptions can look like:
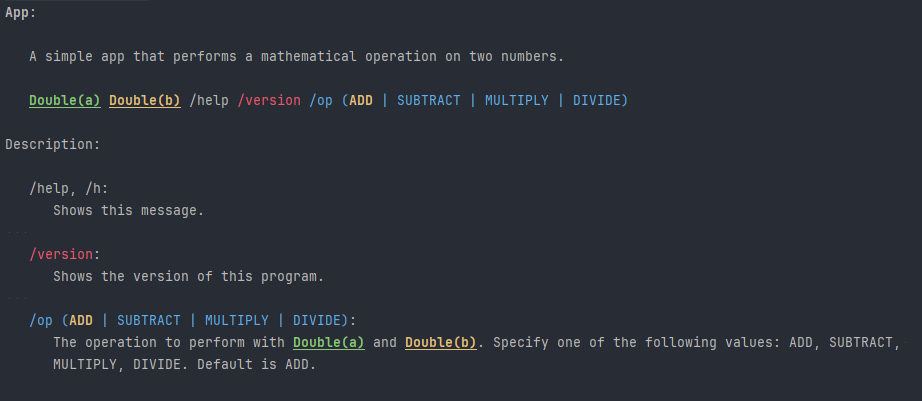
As you can see, the description for the /op
argument contains especial formatting referencing the other arguments in the command. This is done by using description tags, which we will cover in the next section.
Help Message Generation
The heart of the help message generation is the HelpFormatter
class. An instance of this class is created for each command and is responsible for generating the help message for that command.
You can access this instance in cmd.getHelpFormatter()
. When help is shown for a command, this is basically what is being called (cmd.getHelp()
):
The reference of the command passed to the generate
method is what is used to display all the information about the command and its arguments.
Help Formatter
The help contents are generated by a structure of string generators that, when receiving a command, will generate some string representation for it. These are LayoutItem
s.
When a HelpFormatter
is instantiated, by default it will have a set of layout items set (which generate the default help message you see). Here's the default implementation of initLayout
in HelpFormatter
:
As you can see, defining layout items is as simple as creating a new instance of LayoutItem
and passing a generator to it. You can also set the title, indent, and margin for each layout item.
Layout Items
A layout item is basically just a function that receives a command and returns a string. Here's an example of a simple layout item that shows the number of arguments in a command:
Since we are calling addLayoutItems
, our new layout item will be added to the end of the layout list. Here's what is displayed when we call cmd.getHelp()
(excluding the rest of the help message):
The function specified doesn't explicitly need to comply with Function<Command, String>
. It can also be a Supplier<String>
or just a String
.
Default generators
The generators that are used in the default layout items are located in the LayoutGenerators
class.